Git Summary
git Process and command
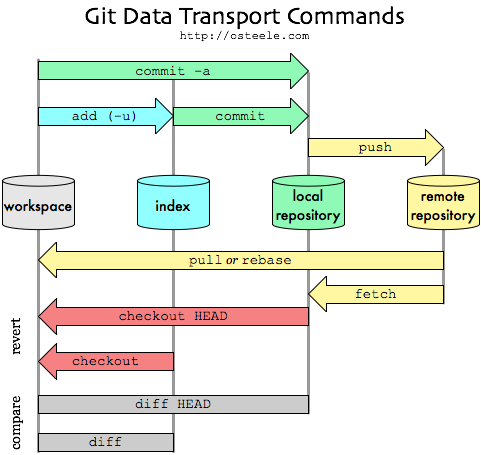
Set configuration
$ git config --global user.name "{github username}"
$ git config --global user.email "{github email address}"
$ git config --global core.editor "vim"
$ git config --global core.pager "cat"
$ git config --list
- optional:
$ git config --global alias.lg "log --color --graph --pretty=format:'%Cred%h%Creset -%C(yellow)%d%Creset %s %Cgreen(%cr) %C(bold blue)<%an>%Creset' --abbrev-commit"
First Repo
$ mkdir first-repo && cd first-repo
$ git init
$ git remote add origin https://github.com/{username}/{reponame}.git
$ touch README.md
$ git add README.md
$ git commit -m "docs: Create README.md"
$ git push -u origin master # setting up stream
Basic workflow of git
$ git status
$ git add .
$ git commit
$ git push origin master
Commit Convention
- 커밋 제목은 50자 이내
- 제목과 내용사이 한칸
- prefix를 사용하여 한눈에 커밋의 용도를 알기 쉽게 한다
- feat: features
- docs: documentations
- conf: configurations
- test: test
- fix: bug-fix
- refactor: refactoring
- perf: Performance
vim key
- A: append text at the end of the string
- o: add line below
- O: insert line above
Using Hexo: Blog posting
Dependencies
- git
- node.js
npm install -g hexo-cli
Init Blog
$ npm install hexo-cli -g
$ hexo init blog
$ cd blog
$ npm install
$ hexo server
New Post
$ hexo new post "{file name}"
$ vi source/_posts/{file name}
$ `jot something`
Generate, test and deploy
$ hexo clean && hexo generate
$ hexo server // local run and check whether the article is generated and displayed well.
$ hexo deploy